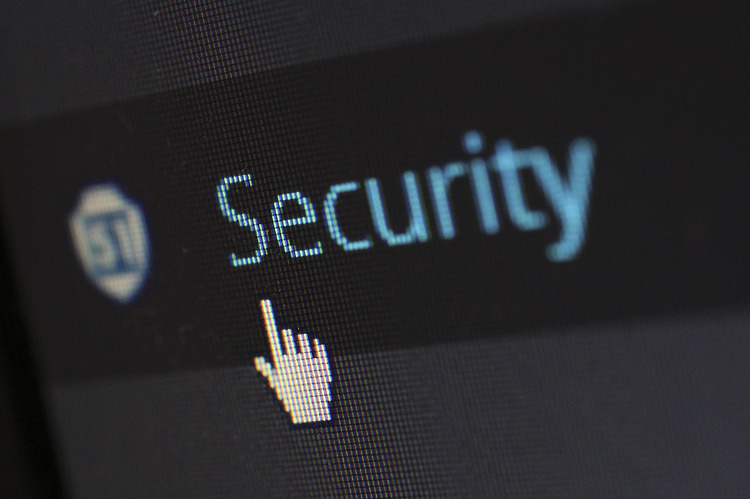
AI, NestJS에서 이메일 인증 방식 구현하기
익스랩 최고 관리자
·2023. 3. 14. 12:11
@nestjs-modules/mailer 사용하기
우선, application에서 이메일 메시지를 쉽게 보낼 수 있는 NestJS용 타사 메일러 모듈을 사용합니다.
SMTP, SendGrid 및 Mailgun을 포함한 다양한 이메일 공급자를 지원하고 이메일 작성 및 전송을 위한 간단한 API를 제공합니다.
1. 설치
npm install --save @nestjs-modules/mailer
2. app.module.ts 파일 구성
import { Module } from '@nestjs/common';
import { MailerModule } from '@nestjs-modules/mailer';
@Module({
imports: [
MailerModule.forRoot({
transport: {
host: 'smtp.example.com',
port: 587,
secure: false,
auth: {
user: 'username',
pass: 'password',
},
},
}),
],
})
export class AppModule {}
3. 서비스 클래스에 MailService 삽입
import { Injectable } from '@nestjs/common';
import { MailerService } from '@nestjs-modules/mailer';
@Injectable()
export class MyService {
constructor(private readonly mailerService: MailerService) {}
async sendEmail() {
await this.mailerService.sendMail({
to: 'recipient@example.com',
subject: 'Test email',
text: 'Hello, world!',
});
}
}
이것이 NestJS 애플리케이션에서 @nestjs-modules/mailer 모듈을 사용하는 방법에 대한 기본 개요입니다. 모듈의 기능 및 구성 옵션에 대한 자세한 내용은 https://docs.nestjs.com/techniques/email에서 공식 문서를 확인하세요.
인증 코드 전송 AuthService 구현
이메일로 인증 코드를 보내고 NestJS에서 확인하는 AuthService 클래스를 구현합니다.
// auth.service.ts
import { Injectable } from '@nestjs/common';
import { JwtService } from '@nestjs/jwt';
import { UsersService } from './users.service';
import { MailerService } from '@nestjs-modules/mailer';
@Injectable()
export class AuthService {
constructor(
private usersService: UsersService,
private jwtService: JwtService,
private mailerService: MailerService,
) {}
async validateUser(email: string, password: string): Promise<any> {
const user = await this.usersService.findByEmail(email);
if (user && user.password === password) {
const { password, ...result } = user;
return result;
}
return null;
}
async sendVerificationCode(email: string): Promise<void> {
const code = Math.floor(Math.random() * 10000).toString();
await this.mailerService.sendMail({
to: email,
subject: 'Verification code',
text: `Your verification code is ${code}`,
});
await this.usersService.saveVerificationCode(email, code);
}
async confirmVerificationCode(email: string, code: string): Promise<boolean> {
const savedCode = await this.usersService.getVerificationCode(email);
if (code === savedCode) {
await this.usersService.clearVerificationCode(email);
return true;
}
return false;
}
async login(user: any) {
const payload = { email: user.email, sub: user.id };
return {
access_token: this.jwtService.sign(payload),
};
}
}
'sendVerificationCode' 메서드는 이메일 주소를 가져와 임의의 4자리 코드를 생성합니다. 코드와 함께 사용자에게 이메일을 보내고 usersService를 사용하여 데이터베이스에 코드를 저장합니다.
* confirmVerificationCode
이메일 주소와 코드를 입력으로 받습니다. userService를 사용하여 데이터베이스에서 저장된 코드를 검색하고 입력 코드와 비교합니다. 일치하면 데이터베이스에서 저장된 코드를 지우고 'true'를 반환, 그렇지 않으면 'false'를 반환합니다.
* saveVerificationCode
UserService 클래스의 일부로 간주되며 확인 코드를 데이터베이스의 사용자 레코드에 저장합니다.
다른 이메일 공급자를 사용하거나 다른 데이터베이스를 사용하여 확인 코드를 저장하는 등 특정 요구사항에 맞게 이러한 방법으로 구현할 수 있습니다.
확인 코드 저장 검색 UserService 구성
다음은 확인 코드를 저장하고 검색하는 UserService 입니다.
// user.service.ts
import { Injectable } from '@nestjs/common';
import { User } from './user.entity';
@Injectable()
export class UsersService {
private readonly users: User[] = [];
async findByEmail(email: string): Promise<User | undefined> {
return this.users.find((user) => user.email === email);
}
async saveVerificationCode(email: string, code: string): Promise<void> {
const user = await this.findByEmail(email);
if (user) {
user.verificationCode = code;
} else {
const newUser = new User();
newUser.email = email;
newUser.verificationCode = code;
this.users.push(newUser);
}
}
async getVerificationCode(email: string): Promise<string | undefined> {
const user = await this.findByEmail(email);
return user ? user.verificationCode : undefined;
}
async clearVerificationCode(email: string): Promise<void> {
const user = await this.findByEmail(email);
if (user) {
user.verificationCode = undefined;
}
}
}
이 예제 구현에서는 메모리 내 배열을 사용하여 사용자 레코드를 저장합니다. 실제 애플리케이션에서는 데이터베이스를 대신 사용할 가능성이 높습니다.
* findByEmail
지정된 이메일 주소가 있는 사용자 객체를 반환합니다. (있는 경우)
* saveVerificationCode
확인 코드를 사용자 레코드에 저장하여 지정된 이메일 주소로 아직 존재하지 않는 경우 새 사용자 객체를 생성합니다.
* getVerificationCode
지정된 이메일 주소를 가진 사용자의 저장된 확인 코드를 검색합니다.
* clearVerificationCode
지정된 이메일 주소를 가진 사용자의 저장된 확인 코드를 지웁니다.
다른 데이터베이스를 사용하거나 사용자 엔티티에 추가 필드를 추가하는 등 특정 요구 사항에 맞게 이러한 메서드의 구현을 사용자 지정할 수 있습니다.
'IT 언어 연구소' 카테고리의 다른 글
VS Code 코딩 보조용 AI 비교: Claude vs ChatGPT vs Gemini (2) | 2025.05.26 |
---|---|
웹앱 개발을 위한 모든 종류의 파비콘과 메타태그 정리 (0) | 2023.04.25 |
AI, Nestjs에서 Cron() 사용 법 (0) | 2023.03.08 |
AI, nestjs의 Task Scheduling 활용하기! (0) | 2023.03.03 |