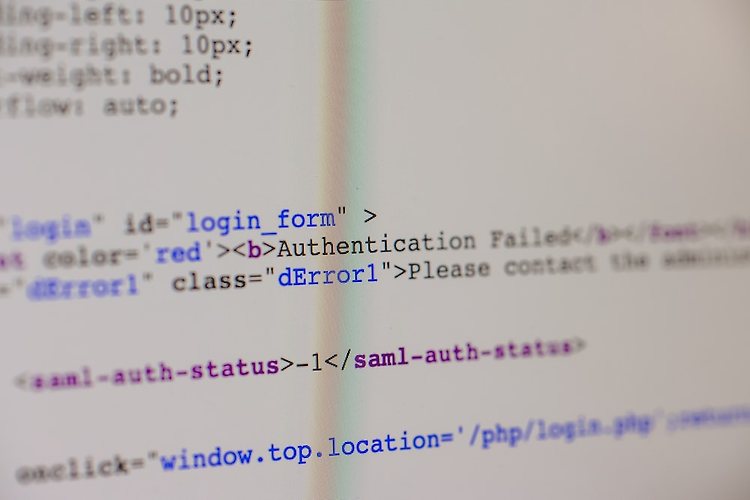
[vue] 'Cannot read property 'x' of undefined' 오류 해결하기
익스랩 최고 관리자
·2023. 7. 19. 11:20
'Cannot read property 'x' of undefined'와 같은 오류 메시지를 마주할 수 있습니다.
이 오류는 객체의 속성을 읽으려고 할 때 해당 객체가 'undefined'로 정의되어 있을 때 발생합니다.
1. 'Cannot read property 'x' of undefined' 오류 예시
이 오류는 객체의 속성을 읽으려고 할 때 해당 객체가 'undefined'로 정의되어 있을 때 발생합니다. 아래의 예시 코드를 살펴보겠습니다.
<template>
<div>
<p>{{ user.name }}</p>
</div>
</template>
<script setup>
const user = {
name: 'John',
age: 25
};
console.log(user.address.city);
</script>
위 코드에서는 'user' 객체의 'address' 속성이 정의되지 않았기 때문에 'Cannot read property 'city' of undefined' 오류가 발생합니다. 이러한 오류를 해결하기 위해서는 아래에서 안내하는 방법을 활용할 수 있습니다.
2. 오류 해결 방안
'Cannot read property 'x' of undefined' 오류를 해결하는 방법은 다음과 같습니다.
가) 'ref' 함수를 사용하여 객체 초기화하기
<template>
<div>
<p>{{ user.value.name }}</p>
</div>
</template>
<script setup>
import { ref } from 'vue';
const user = ref({
name: 'John',
age: 25
});
console.log(user.value.address?.city);
</script>
위 코드에서는 'ref' 함수를 사용하여 'user' 객체를 초기화하였습니다. 'ref' 함수를 사용하면 객체를 'value' 속성을 통해 접근할 수 있습니다. 또한, 선택적 체이닝 (Optional Chaining) 연산자인 '?.'을 사용하여 'address' 객체와 'city' 속성의 존재 여부를 확인할 수 있습니다.
나) 조건문을 활용하여 객체 확인하기
<template>
<div>
<p>{{ user.name }}</p>
</div>
</template>
<script setup>
const user = {
name: 'John',
age: 25
};
if (user.address && user.address.city) {
console.log(user.address.city);
} else {
console.log('주소 정보를 찾을 수 없습니다.');
}
</script>
위 코드에서는 조건문을 사용하여 'user' 객체와 'address' 객체, 그리고 'city' 속성의 존재 여부를 확인합니다. 객체와 속성이 모두 정의되어 있을 때에만 'console.log()' 문이 실행되고, 오류를 방지할 수 있습니다.
'Cannot read property 'x' of undefined' 오류 해결 방법의 장단점
이러한 방법들은 'Cannot read property 'x' of undefined' 오류를 해결하기 위해 효과적입니다. 그러나 각각의 방법은 장단점을 가지고 있습니다.
'ref' 함수를 활용하는 방법은 코드를 간결하게 유지할 수 있고, 선택적 체이닝 연산자를 사용하여 코드의 가독성을 높일 수 있습니다. 그러나 'ref' 함수의 사용은 객체에 접근할 때마다 'value' 속성을 붙여야 한다는 불편함이 있을 수 있습니다.
조건문을 활용하는 방법은 코드를 명시적으로 작성할 수 있으며, 객체와 속성의 존재 여부를 직접 확인할 수 있습니다. 그러나 복잡한 구조를 다룰 때에는 조건문이 중첩될 수 있고, 코드의 가독성이 낮아질 수 있습니다.
'IT 언어 연구소 > Error 관리' 카테고리의 다른 글
RangeError: Maximum call stack size exceeded 오류 원인과 해결책 (0) | 2023.07.25 |
---|---|
TypeError: Cannot read property '...' of null/undefined 원인과 해결 방안 (0) | 2023.07.24 |
Indicates a value that is not in the set or range of allowable values 원인과 해결 방안 (0) | 2023.07.24 |
Uncaught TypeError: Cannot read property 'value' of undefined (0) | 2023.07.23 |
[Vue] Function statements require a function name (0) | 2023.07.22 |